limit <value>
does indeed limit the overall result set, but could be applied both before and after the group by
clause. Sadly, it didn’t produce the wanted result. Since we’re dealing with tasks, I’m not quite sure how to only fetch the first task from each file, maybe @mnvwvnm could do that, but I’m not enough of a wizard to do that for a straight DQL query.
However, I do know a little javascript, and since I lead you onto this path, I would like to present some alternatives using DataviewJS, which is getting to be my new favorite toy.
## Postprocessing magic
```dataviewjs
let firstTasks = []
const tasks = dv.pages('"ForumStuff/f48291"')
.file.tasks
.where(t => !t.completed)
let prevPath = ""
for (let task of tasks) {
// console.log(task)
if (task.path != prevPath) {
firstTasks.push(task)
prevPath = task.path
}
}
dv.el("h3", "With no project references")
dv.taskList(firstTasks, false)
dv.el("h3", "With project references")
dv.taskList(firstTasks)
```
## Even more magic
```dataviewjs
let firstTasks = []
const tasks = dv.pages('"ForumStuff/f48291"')
.file.tasks
.where(t => !t.completed)
let prevPath = ""
for (let task of tasks) {
// console.log(task)
if (task.path != prevPath) {
task.text = task.link + ": " + task.text
// task.text = task.link.fileName() + ": " + task.text
firstTasks.push(task)
prevPath = task.path
}
}
dv.el("h3", "Inline project reference")
dv.taskList(firstTasks, false)
```
These two (and a half) scripts, produces three variants of the output which all lists just the first uncompleted tasks from my two example projects, aptly named FirstProject
and Second Project
.
The output produced are:
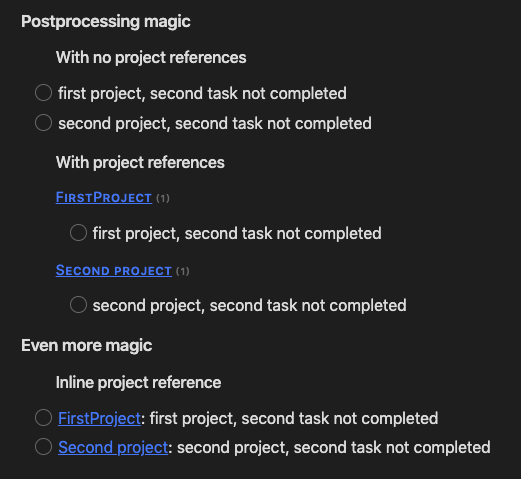
My two example files are simply (with “First” changed to “Second” in the other file):
- [x] First project, first task completed
- [ ] first project, second task not completed
- [ ] first project, third task not completed
- [x] first project, fourth completed task?!
Some explanations are in order
There are just slight variations between the scripts, and they both use the same principle:
- Collate all tasks that are not completed, and store these in
tasks
- Loop through all tasks, and store away the first task in each file
- Present the tasks using
dv.taskList()
The differences are how we present the result. The first variant presents just the task (due to the false
at the end of the dv.taskList()
). Do note that as usual the task text links to the original note/project, but it can be kind of hard to identify the project.
So the second variant includes the note header, which I personally don’t like that much since it introduces another element into the list, and especially when we only present one task, I think it becomes a little cluttered.
Therefor I made the third variant, where I actually change the task text, and include the link to the original note. If you look carefully in the code, you’ll also see commented line with just the fileName, if you don’t like the visual link. This I think looks the best, as it indicates which project the task originates from.
So, hopefully this helps, and if lucky a simpler pure query will present itself in the near future. But until then, this could be an option to get the first uncompleted task from your projects.