That’s a great idea! I’ve used it to improve my new note template.
Here’s how you do the split:
const file = app.vault.getAbstractFileByPath('path/to/new_note.md')
// Create the new leaf
const newLeaf = this.app.workspace.getLeaf('split')
await newLeaf.openFile(file)
// Set the view to edit mode
const view = newLeaf.getViewState()
view.state.mode = 'source'
newLeaf.setViewState(view)
// Give focus to the new leaf
this.app.workspace.setActiveLeaf(newLeaf, { focus: true })
// Move the cursor to the end of the new note
this.app.workspace.activeLeaf.view.editor.setCursor({line: 999, ch: 0})
And here’s my full template which does all 5 of your steps. This is the template I use for all my new notes, with the new addition of the split (which I really like!):
async function main() {
const tp = app.plugins.plugins['templater-obsidian'].templater.current_functions_object
tp.user._init_template(this)
const templates = [
{
label: 'Default note',
template: 'Templates/Default note'
},
{
label: 'Project',
template: 'Templates/Project',
destination: '05 Project management/Projects'
},
{
label: 'Person',
template: 'Templates/Person note',
destination: 'People',
title: '👤'
}
]
// Present the list of templates to choose from
const chosen = await this.tp.system.suggester(templates.map(x => x.label), templates)
if (!chosen) return ''
const name = (await this.tp.system.prompt('Name for the new file:', chosen.title || '')) || moment().format('YYYY-MM-DD HH.mm.ss')
const addLink = await this.tp.system.prompt('Insert link in the current file?', 'Enter for yes or Escape for no')
const destination = chosen.destination || this.tp.file.folder(true)
const res = await this.createFromTemplate(chosen.template, name, destination, !addLink)
if (addLink) {
// Open the new file in a pane to the right
const file = app.vault.getAbstractFileByPath(`${destination}/${name}.md`)
// Create the new leaf
const newLeaf = this.app.workspace.getLeaf('split')
await newLeaf.openFile(file)
// Set the view to edit mode
const view = newLeaf.getViewState()
view.state.mode = 'source'
newLeaf.setViewState(view)
// Give focus to the new leaf
this.app.workspace.setActiveLeaf(newLeaf, { focus: true })
// Move the cursor to the end of the new note
this.app.workspace.activeLeaf.view.editor.setCursor({ line: 999, ch: 0 })
}
return res
}
module.exports = main
It’s written in Templater “Users script” format, so you’ll need to put it in your users script folder, and then call it from a normal template like this:
<% tp.user.newNote(tp) %>
Assign that template to a hotkey like Ctrl + N, for new note.
When you run it, you’ll be presented with a list of new note templates, which will be created in the appropriate folder and with the appropriate content:
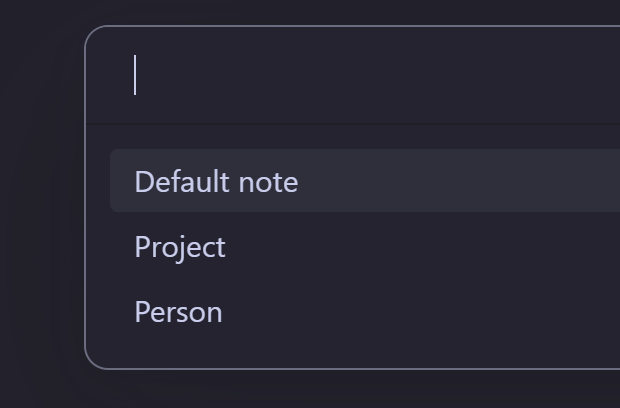
You can chose whether to add a link into the current file, which will open up the split to the right, or you can simply open into the new note without adding a link in the current file: